Hello! I’m a developer of the LangJournal app. Today, I want to share more about the newly added video posting and playback features in our app.
LangJournal is an app that allows you to learn languages through diary entries. Until now, we could only post images, but we felt that the video posting feature was absolutely necessary, so we decided to add it.
We used React Native and Expo to implement this feature. The main steps are as follows:
- Use of expo-image-picker: Allows users to select videos from their device. This library provides an easy way to access the gallery on your smartphone and select media.
- Use of expo-av: Implements the playback function for the selected videos. expo-av is optimized for the playback of video and audio content. It also offers features like fast-forwarding and pausing, making implementation easier.
- Use of expo-video-thumbnails: Generates thumbnails for videos. Saving thumbnails is convenient for use in list screens, as it eliminates the need to retrieve videos every time.
- Use of Firebase: Saves both the thumbnails and the videos themselves to Firebase. We chose Firebase for its compatibility with Expo and its rich library resources.
This process involves users selecting a video and uploading it to Firebase.
import * as ImagePicker from 'expo-image-picker';
import { storage } from 'firebase';
const pickVideo = async () => {
let result = await ImagePicker.launchImageLibraryAsync({
mediaTypes: ImagePicker.MediaTypeOptions.Videos,
});
if (!result.cancelled) {
const videoUri = result.uri;
const response = await fetch(videoUri);
const blob = await response.blob();
const videoRef = storage().ref().child(`videos/${new Date().getTime()}`);
await videoRef.put(blob);
generateThumbnail(videoUri, videoRef);
}
};
Generates a thumbnail from the selected video and uploads it to Firebase. This process makes the app’s list display and previews faster and more efficient.
import { createThumbnailAsync } from 'expo-video-thumbnails';
const generateThumbnail = async (videoUri, videoRef) => {
try {
const { uri: thumbnailUri } = await createThumbnailAsync(videoUri);
const response = await fetch(thumbnailUri);
const blob = await response.blob();
const thumbnailRef = storage().ref().child(`thumbnails/${videoRef.name}`);
await thumbnailRef.put(blob);
} catch (e) {
console.warn(e);
}
};
We use expo-av for playing videos within the app.
import { Video } from 'expo-av';
const VideoPlayer = ({ videoUri }) => (
<Video
source={{ uri: videoUri }}
rate={1.0}
volume={1.0}
isMuted={false}
resizeMode="cover"
shouldPlay={false}
useNativeControls
style={{ width: 300, height: 300 }}
/>
);
- Usability: We made it easy for users to select and upload videos.
- Performance: The uploading of videos and thumbnails is done efficiently. Even with large file sizes, Firebase’s fast upload feature ensures that users are not kept waiting.
- Data Management: Using Firebase to save videos and thumbnails simplifies data management. This ensures that the videos and thumbnails uploaded by users are securely stored and easily accessible within the app.
The video posting and playback feature in the LangJournal app provides a new way for users to record and share their language learning experiences. Please download the LangJournal app and try out this new video posting feature!
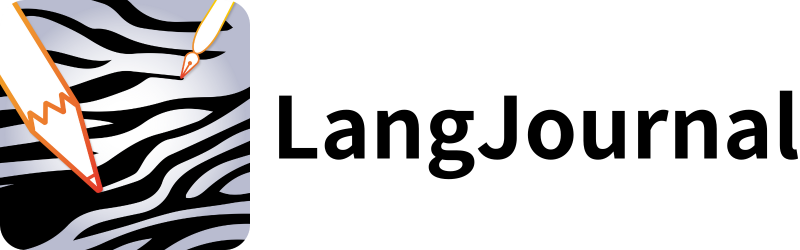
LangJournalは、日記を書くことで英語やフランス語などの外国語を学べるアプリです。英語学習に興味がある方や、私が開発したこのアプリに関心を持っている方は、ぜひインストールしてお試しください。
LangJournalのサイトはこちら